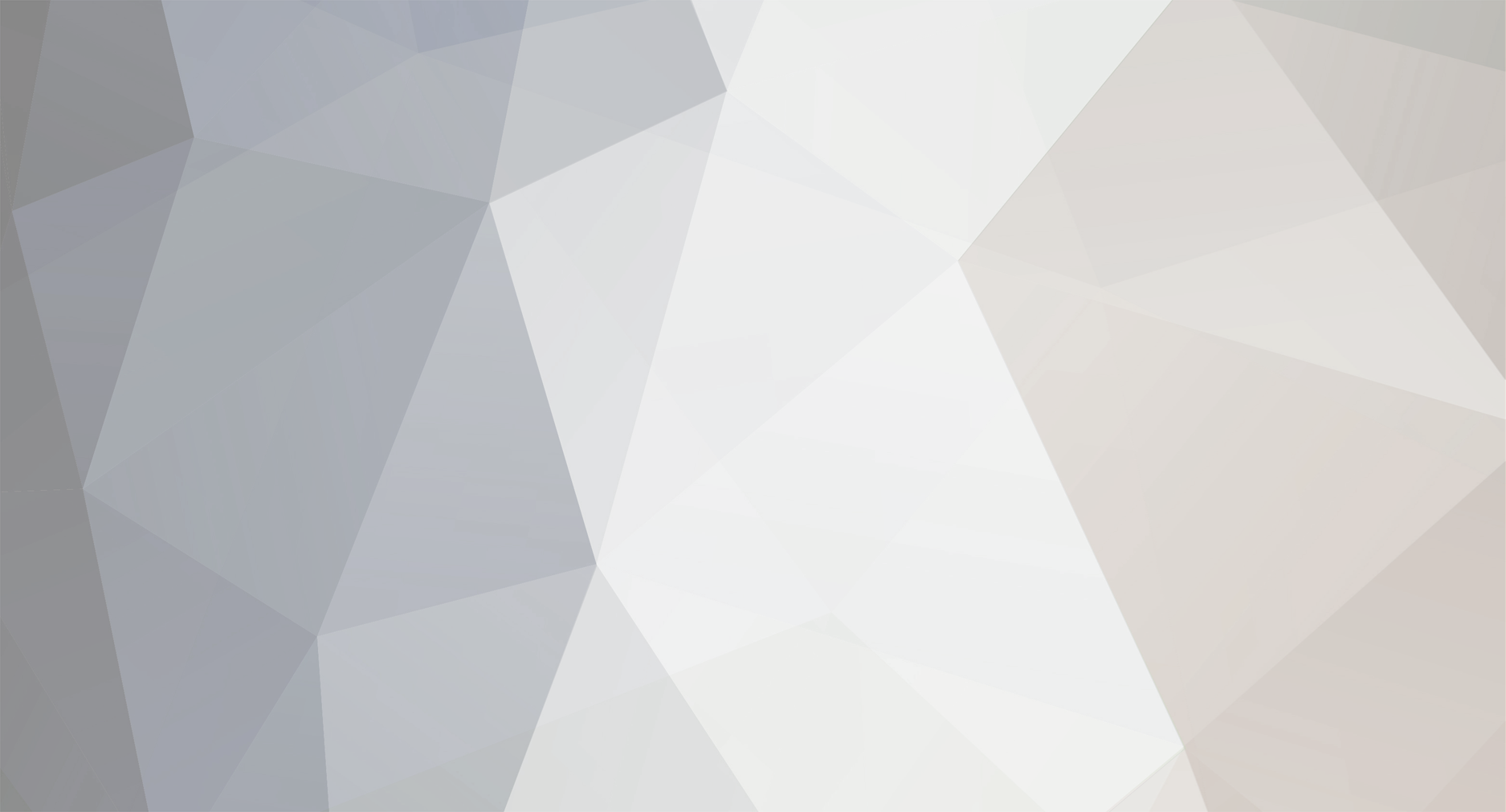
fyhuang
-
Posts
838 -
Joined
-
Last visited
Posts posted by fyhuang
-
-
I have a feeling that you might need to update your DirectX version, drivers, or even Windows version. The site does not list minimum requirements, and it doesn't look like quite a professional site to begin with. I suppose I'm not gonna stop you from using it, but just to be sure, update your drivers and DirectX and see if it helps.
Cheers!
-
However unlikely this may be, replacing Apache's tray code with their own code has the ABSOLUTE SMALLEST POSSIBILITY of introducing a security flaw
. But that's just being paranoid, you'll be fine
.
I just don't like the abusive use of gradients in that menu. Gradients are amazing IF YOU USE THEM RIGHT but most people just abuse gradients to the extreme cause it 'looks cool'... that image is annoying too.
Cheers!
-
Whoa. I thought by minimize to desktop, you mean like Unix-style becoming Icons on the desktop. That would've been cool.
But otherwise, I am totally at a loss to why your system did that. As it seemed back when SP2 was released - everyone's system'll act differently, and many would act more negatively than others
. I'm not sure what I can do for you... if it is possible to uninstall SP2, do so. Switch to Linux. Reinstall Windows. Whatever you want, but it doesn't look like your computer's gonna want you to install SP2 again... at least, not without a Windows reinstall.
Sorry I can't help much more, my computer didn't react to SP2 (violently), and I'm on a Linux now (it's been a while...).
Cheers!
-
I suppose this is good for you Windows users but us Linux users have it easy
.
Cheers!
-
Wait a second. I had this problem when I was installing my SuSE Linux - at first I thought it was a software problem, then I found out I had a faulty hard disk. I dunno why that would affect your Live-CD's speed though.
Heating could also be a problem (although my P4's 'thermal protection' is kinda not working - when it overheats, it just stays at 3.0 GHz and does nothing to protect itself. Linux does issue a warning message though, but like I said, nothing happens to my speed).
Cheers!
-
You can make bootable CDs from *any* distribution, but generally that requires a lot of Linux experience.
However, if you're interesting in trying other live-distros of Linux, the Amarok CD, which has the Amarok music player, KDE, and a bunch of songs. Or, the SuSE LiveCD. Get the SuSE 9.2 CD (GNOME or KDE, whatever your preference) if you only have a CD-burner, and the 9.3 CD if you have a DVD-burner and blank DVDs.
Amarok Live-CD: http://amarok.kde.org/wiki/index.php/AmaroK_Live
SuSE 9.2 live: ftp://mirrors.usc.edu/pub/linux/distribut...86/live-cd-9.2/
SuSE 9.3 live DVD: ftp://mirrors.usc.edu/pub/linux/distribut...eDVD-i386-1.iso
I personally use SuSE Linux at home (the installed version, not live-CD), and I must say it's a great Linux distribution, even if they focus more on KDE than GNOME (KDE is fine by me but it's way too resource-hoggy, slow-loading, and it suffers from a bad case of featuritis).
Cheers!
[edit] Fixed links
-
Huh, for me MSN is getting less reliable than AIM now (which says something
), it logs me off about once every now and then, then my client re-logs me on. It's a little irritating but suggests bad things about the MSN network.
It could, of course, just be my client (go gaim!). But, with your comments I think it's Microsoft's problem.
Cheers!
-
No, getting it installed isn't that hard... it's the stability and bug problems that hit most Linux users...
Not to mention their OpenGL version is so far behind (nVidia's got OpenGL 2.0 now while ATi is still at 1.3), and like I said - stability. For me, Blender, NWN were the two most common programs that crashed, but everything else crashed sometimes too.
Cheers!
-
The pound sign, and generally anything that's not used in American English, is not really a part of the standard ASCII character set. If you want to use international symbols, use Unicode. Unicode is not possible (AFAICT) with 'standard' C, you have to use the Win32 API or something like Pango.
HTH!
-
ATI Mobility Fire GL V3200 128MB memory
Noooo! NOOOOOO!!!
Keyboard: Full size AzertyYes! (although I prefer QWERTY, full-sized keyboards are *very* rare on laptops, even the big huge bulky ones from Toshiba have mini-keyboards)
only has a 1024x768 resolution and 855 shared videoI don't see what's wrong with 1024x768, especially on such small screens as laptop screens (I can't stand anything above 1024 on my 19" desktop monitor, I think it's too straining on my eyes). 855 shared video, that's Intel right? Intel might be slow but ATi is completely useless if you're planning on anything besides Windows and DirectX. ATi OpenGL support, even on Windows, is very bad. And that control center app is completely stupid. The ATi's not mainstream either, it's a workstation card.
If you think you can risk voiding your warranty (not sure about this, check the warranty when you get it), you can replace the ATi card with something better from nVidia (nVidia beats out ATi in the low-end new gfx card market (i.e. X300 vs. 6200), at least IIRC).
Cheers!
-
It's probably that the MSN network is down for a bit. If your normal Internet works and you haven't made any changes to your MSN client, just wait around for a bit and see if it helps. If not, come back
.
Hope it helps!
-
I tried it and it didn't work for me (then again, nothing works correctly on Windows)...
-
There is no 'standard' number of processes that a computer should run. It really depends on what you have installed and all that. It also varies depending on OS (i.e. Win98 has very few processes, WinXP has a lot more, and Linux has more than you can count - I have 192 right now).
Do as Desmond says and you should be fine. Beware though, changing settings in msconfig will cause your computer to emit an (annoying) message every time it boots, which can be changed somewhere in the registry (but I don't remember exactly where)...
Cheers!
-
The iPod shuffle does indeed have one of the highest sound qualities in the pertable music player market out there, but its completely 'jukebox-mode' and if you ask me, the headphones make much more of a difference. When you're on a loud train or jogging or whatever there's going to be so much background noise that it will matter more that your earphones block outside noise than your player make better noise.
Cheers!
-
Most companies can't afford to have customers pay $0.99 - they're losing money actually. IIRC iTunes only broke even last year...
What frustrates me is all these competing music stores but none that work with Linux or Mozilla... (the 'preview' option on all the music stores I've seen require IE to work)
Cheers!
-
I love the Creative Zen Micro but it's not exactly 10+ GB of memory...
-
And, nVidia released Linux drivers that support 7800 the day it came out. That's pretty good
.
I just recently got a 6600, and I must say, it was cheap and performs really well too. I get like 45 FPS in DOOM 3!!! At medium quality, 800x600! That's pretty amazing stuff
.
Cheers!
-
Most people don't even know that Firefox exists. Even if they do, they're like, Firefox is better than IE and that "Mozilla" stuff isn't...
-
Typewriter... bleh. What a bad monospace font. I'm looking for a monospace font that's nice and smooth (kind of like a Sans Serif font but monospaced?)... like the ones they use in some technical books (see Code Complete, 2nd ed., Microsoft (!) Press). I need to find that font for my text editor...
Cheers!
-
I have Halo, I think it's a good game, but it's not the perfect game that everyone else says it is. I have Halo for PC - it's primarily built for Xbox. Therefore, fairly small levels (in-between loading screens that is), extremely overpowered graphics for low framerates, etc. etc.
UT2004 looks better IMHO and runs at at least 3x the FPS.
Anyhow, Halo is well-polished, well-made, but is definitely not the best game in the world. Too overrated, yes. Game-review sites were probably just trying to get Microsoft's favor when they reviewed it so sky-high. On the other hand, not many games so far have recieved the kind of polish and coherence that Halo has. While some levels are confusing (the swamp one got me stuck for hours cause I couldn't figure out where to go
), overall it's a good game.
I wouldn't buy Halo 2 however, everyone says it's like, oh better graphics (actually it's just darker and Master Chief is shinier - the Xbox can't handle much more than Halo graphics), oh better AI (adding more cases to a switch statement for those programmers out there? I mean, yeah sure they can duck better now - does that mean the AI is better? or just that the programmer tweaked the ducking code? everything's hardcoded...). And then, here's the big one - more weapons! God dammit, what's up with that? Every time gamers see a new FPS, they're looking to see how many weapons it has. That's just stupid.
Besides, Halo had an amazing story and I'm not sure about how much Halo 2 can continue on that. Sequels almost always have worse stories because the first game had already offered closure to that story, and then the sequel introduces something completely new, making it a different game altogether...
My (long) .02. Cheers!
-
I was actually thinking about ways to encrypt songs with effective, foolproof DRM, but I haven't yet thought of anything that would work every time. Even if it is encrypted, it is necessary to store the encryption key along with the song so that it can be played legitimately. Hacked players don't need to check the DRM settings first, they'll just go right ahead and decrypt it.
Now, what if you stored the key on the Internet and encrypted each downloaded song with a different key? The person would have to have an internet connection to play the song, which makes it completely ineffective for non-networked devices (i.e. digital music players) and a big nuisance for dial-up and other non-persistent connection users.
See what I mean? DRM is useless. Get with the open-source program
!
Cheers!
-
While big brands do get more negative recognition smaller brands can do even worse. I would personally stay away from Fujitsu, merely because they don't have much of a computing track record (from what I've seen and heard).
And while paying more doesn't always mean you get more, it does make the difference between a 'value' laptop (dirt cheap!) and a 'workstation' laptop (not so dirt cheap but much more reliable!).
Cheers!
-
They have not yet seen the wonders of middle-clicking...
Seriously though, only a small percentage of people actually like Internet Explorer - everyone else isn't aware enough to make the switch.
Cheers!
-
IBM is high-quality, enterprise-grade laptops. I highly doubt any manufacturer has yet matched their quality, especially such a small brand as Fujitsu. I know no one with a Fujitsu laptop.
If you have had bad experiences with a past laptop, my advice would be to spend more money on it. You'll be assured that you will get what you paid for, in a manner of speaking. Your laptop won't suffer from as many manufacturing/quality defects and you'll be better off with it.
My other advice - stick with the brands that everyone else uses. While the majority is not always right (as is the case with, say, HP computers), usually it is good to stick with what is popular (because it'll probably be better, as less people buy a product that suffers from bad quality). If in doubt, consult back here
.
Cheers!
How do you do symbols in C++?
in Computer Desk
Posted
OMG. Is that copied directly from the programming book? Cause that exhibits some very, very bad programming habits...
I'd like to make a few suggestions on that - mainly:
Is firstly, a bit redundant. Why not just:
Or even better, use a switch statement (not sure if you learned these yet). Second, the variable is called menu? It doesn't represent a menu though, it represents the user's selection, so it should be called 'selection' or 'choice'. And third, don't use global variables. There's no point:
And by the way, if you're a beginner and your code has the word 'char' in it, you have a bug
. As for answers to your questions:
1. Since cin doesn't perform error checking, entering a string instead of an integer will produce incorrect results.
2. Same.
3. Integers are limited to a certain range of numbers (an integer can only represent 2^32 numbers). If you enter something in above/below that range, it will overflow. Also, long strings may not be parsed correctly by cin.
The solution to 1 and 2? Write some simple error checking. Check if all the characters in the string are numbers:
Hope that helps (kinda long, but okay!)!