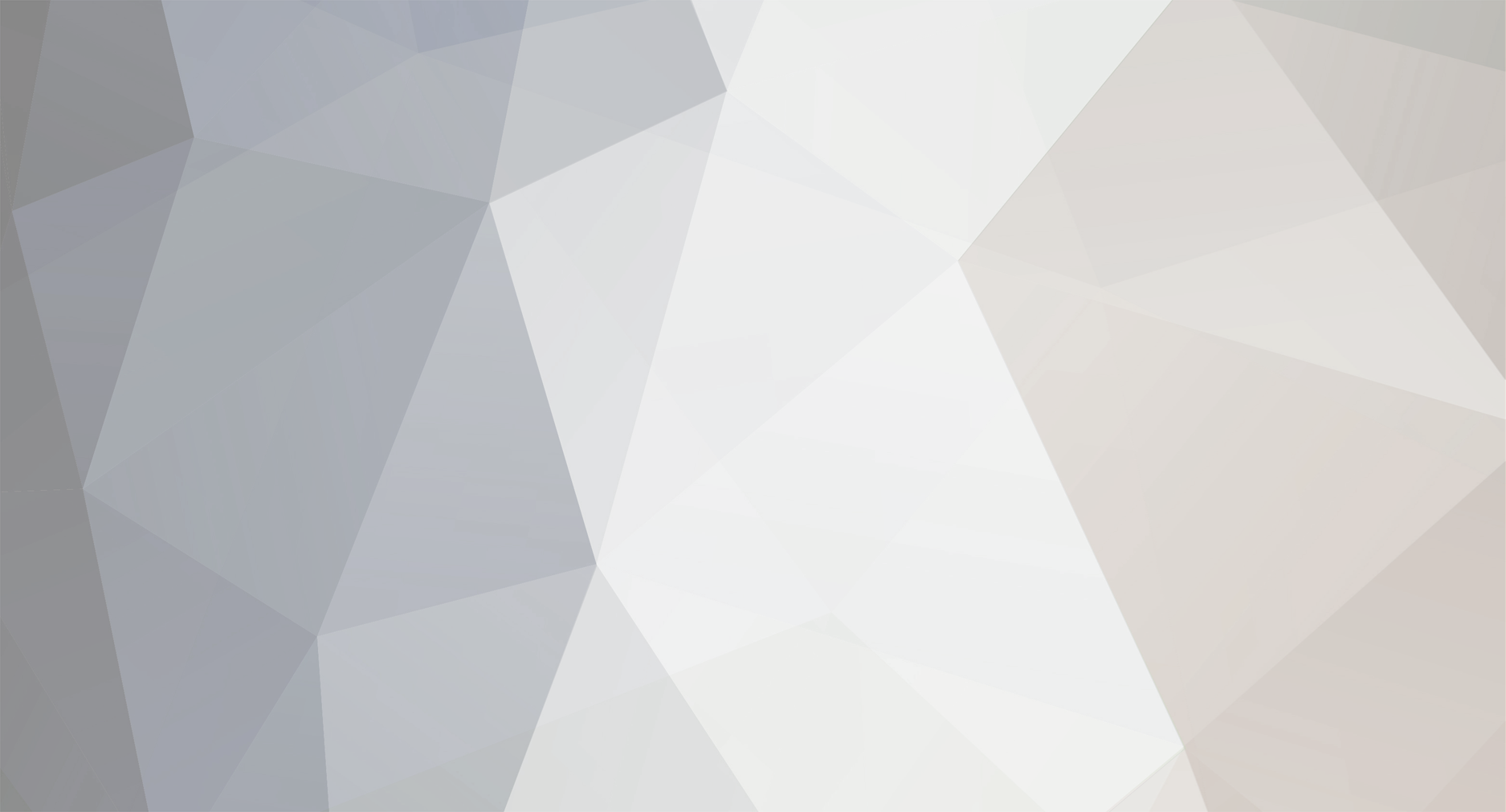
Ykkrosh
WFG Retired-
Posts
4.928 -
Joined
-
Last visited
-
Days Won
6
Everything posted by Ykkrosh
-
AoM-like pathfinding/movement
Ykkrosh replied to Ykkrosh's topic in Game Development & Technical Discussion
Hrm... I've been rethinking this a bit, based on insider information. Experience shows that's not okay, even after trying to optimise the code, so algorithmic changes are needed. What AoM does instead is (I think): When a unit is moving from A to B, start with the proposed path A-B and check it for obstructions. If the first obstruction you hit is C, let C0 be the point of intersection, and let C1/C2/C3/C4 be the vertexes of C (where C1 and C4 are both on the edge you hit). Construct the paths A-C0-C1-C2-C3-C4 and A-C0-C4-C3-C2-C1 (where the parts in bold are the known obstruction-free segment), and repeat this process for each path in parallel. Check C0-C1 for obstructions, discover it hits D, construct the paths A-C0-D0-D1-D2-D3-D4 and A-C0-D0-D4-D3-D2-D1; check C0-C4 for obstructions, discover happily there's no obstruction, so construct the paths A-C0-C4-C3-C2-C1 and A-C0-C4-B. Now there's four paths, so repeat for them all. In general, when the next step of the path hits an obstruction, throw out the remaining steps and construct two paths that try to circle around the obstruction in each direction; when the next step doesn't hit an obstruction, continue circling in that direction and also try to go directly from there to B. If I got the details right (I'm not sure I have quite), then this gives an algorithm that heads towards B and then basically walks around the outsides of any overlapping group of obstructions that it hits until it's reached far enough round the other side to leave the group and carry on towards B. After finding the shortest path by this method, simplify the path. Start at point A, and loop backwards over the waypoints W in the path, and if there's an unobstructed line from A to W then cut out all the intermediate waypoints and go direct from A to W. Otherwise, loop backwards over W and find point P on the line A-C0 such that A-P and P-W are perpendicular; if P-W is unobstructed then go directly along A-P-W and cut out intermediate waypoints. Otherwise, we can't simplify from A, so try again from the next waypoint. I believe this matches the observable behaviour of AoM, including what could be considered bugs. For example, the red circle wants to reach the green cross, and follows the red path: It tries to go direct, hits an obstruction, then loops around the line of obstructions. That's longer than a path that goes around the blue obstruction - our current pathfinder implementation will always find the shortest path, but in this case AoM chooses the longer one. If you remove the blue obstruction, then the path simplification takes effect: the first three down/right/up segments of the path get cut out and it goes straight across to the right. It's possible to get pretty crazy paths if you have a complex layout of overlapping obstructions and then carefully arrange obstructions to block this simplification. About performance: Assume we create at most M extra intersection-vertexes (like C0, D0 in the earlier example) per obstruction, where typically M=1. (This is a bogus assumption - there's no upper limit unless the algorithm explicitly forces it. (In AoM I got a path to create five new intersection-vertexes on the edges of a single wall object, before running out of physical space, so it doesn't impose a visible limit). I'll hand-wave this for now and assume it's a small constant). With N obstructions, we have at most N*(4+M) vertexes. If we process paths in the correct order, I think it's kind of like A* with an admissible heuristic, so we'll only visit each vertex once (since the first time will always be the shortest path through that vertex). So the main loop of the algorithm gets called at most N*(4+M) times, and each time it's checking for intersections with the N obstructions, so that's O(N^2), which is much better than the O(N^3) of the current pathfinder implementation. I'd be surprised if I explained this well enough to make sense to anyone, but I think I can almost understand it myself now. I suppose I should try implementing it some time soon, before I forget it all - in theory it should be much faster than the current code, and it will sometimes pick sub-optimal paths but probably nobody will notice (and it's good enough for AoM (and AoE3 and AoEO, I think)), and the implementation shouldn't be more complex than the current code. The main risk is that I'm not understanding the algorithm correctly and it won't actually work, but the best way to discover that is probably just to do it and see what breaks. -
I think the engine/script interface isn't likely to change much, since it seems to basically work and I'm not sure there's much that needs to be added (except some more events). common-api might change quite a bit depending on what the higher-level scripts want it to do, but I don't have any current plans so it'll depend on feedback and further experimentation. TestBot should probably be pretty much entirely rewritten. If people write AIs now then there's no guarantee of compatibility and they'll probably break at some point; but if people don't write AIs now then we'll have no experience to base the design on so it'll never become stable. So I think now's a good time to try writing some
-
They say: I suppose it makes some sense - the Robot developers are familiar with the Bang engine so they're the best people to get AoEO up and running, for which Microsoft can give them lots of money that can help fund their original projects, and then someone else can come in and do all the dull money-making exploitation of players with premium content (the mentioned Booster Packs and Vanity Packs). (Incidentally, I've always liked orcs, so I don't see why they must die.)
-
Um, slightly late, but uploaded now.
-
Ah, looks like a shadowing problem. If you're able to experiment a bit, maybe you could try using the old drivers and editing source/renderer/ShadowMap.cpp lines 367/368 to both say "GL_NEAREST" instead of "GL_LINEAR" and recompile and see if that makes any difference? (I'd be pleasantly surprised if that actually worked, but it's probably worth a try just in case )
-
Sounds good . I guess there's still lots of things that aren't easily understandable without searching through the code - would some documentation be helpful at this stage? If so, what kinds of things would be most useful to document? (The data that common-api/base.js receives from the engine? The way the simulation components send data to the AI scripts? The way common-api exposes entity data? The way testbot does its stupid broken planning thing? How to get started with writing and running scripts? etc) Same question probably applies here - hopefully documentation would make it easier to do what you want, but what specific things need documenting? (#740 should be fixed now). Sounds great . I don't really like the design of TestBot at all, but I'm not really sure how to design it much better, so it's good for me if someone else can do the work to make a decent AI The implementation is already fundamentally event-based (since that's necessary for performance). The data that the engine sends to the AI scripts every turn looks like: { "entities": { "7683": { "id": 7683, "template": "units/hele_support_female_citizen", "position": [ 97.58279418945312, 73.37493896484375 ], "hitpoints": 75, "owner": 1, "idle": false, "resourceCarrying": [] } "7741": { "position": [ 447.17420959472656, 133.0516815185547 ] }, "8603": { "hitpoints": 72, "position": [ 419.1849822998047, 397.58448791503906 ] }, }, "events": [ { "type": "Attacked", "msg": { "attacker": 8613, "target": 8603 } } ], "map": /* terrain/obstruction data */, "passabilityClasses": /* stuff for interpreting obstruction data */ "players": ..., "timeElapsed": ... } In the "entities" section, 7683 is a new entity (so it includes all the relevant state data for that unit); 7741 is an old unit that has moved since the last turn (so it only sends the new position value - the AI scripts are responsible for remembering the previous turn's entity state and applying these new changes); 8603 is an old unit that has moved and also been attacked (so it has a new position and hitpoints). The "events" section is a list of various events that occurred in the previous turn (currently Create, Destroy, Attacked, ConstructionFinished, TrainingFinished, PlayerDefeated). TestBot uses common-api/base.js to process this data - it maintains the current entity state (in this._rawEntities) and updates it based on the new "entities" data from the engine. Most of the items in the "events" list just get ignored, since TestBot didn't need to use them. If you do want to use them, probably a good approach is to make a copy of common-api and edit base.js to do whatever you want (e.g. maintain a list of event handlers and let the AI subclass set up the handlers in its constructor) and experiment with an event-based API that way - there shouldn't need to be any changes to the engine code, it's all just relatively straightforward scripting. (The goal of common-api is to provide a useful base for all AI scripts, so ideally any successful experiments would get merged back into it; but there's no obligation to use common-api at all, and it's easier to play around with a separate copy to avoid breaking any other AI scripts.) So I think you should be able to do what you want by just editing the scripts. If you need the engine to send more gameplay events then I can probably add those (they just need updates to the component scripts), and if the engine API needs to be document then I can do that; otherwise I think I don't need to be involved (and I'd prefer not to have to be, since I'm still not sure how to do a good event-based AI design so it's better handled by people who believe in it ) I think that players writing 'assistant' scripts is a separate topic - they're more part of the GUI system than the AI system, and there's different requirements on security and performance etc, so the implementation would be completely different. It's an interesting idea (and probably worth discussing more) but I don't think it'd have any effect on the AI design so it's not particularly relevant here. I think my concern is: What would you do with that information? I expect you don't want to send your whole army rushing after a single enemy archer who pinged one of your outpost towers - you probably want to do some kind of threat calculation based on the rate of damage and the concentration of enemy forces, so you're forced back to looping over lists of entities, and you don't want to do all that looping every single time a unit gets injured, so you'll just run the calculation every few seconds, and the event-based system doesn't seem to give any real benefit to this process.
-
I'm not sure what the lighting weirdness would be - could you perhaps post a screenshot? I don't know the current situation is with r600 driver compatibility, but I'd guess the proprietary drivers would work better. The game is probably slow partly because it's compiled in debug mode - assuming it's this package, the PKGBUILD file should say "make CONFIG=Release" instead of just "make", and the run script should use "pyrogenesis" instead of "pyrogenesis_dbg".
-
#684. Until we disable S3TC automatically, you can do it manually by creating a file ~/.config/0ad/config/local.cfg with nos3tc=true Hopefully that'll make things better
-
Yes, there should be binaries/system/pyrogenesis and binaries/system/test. Maybe there was an error during compilation, hidden somewhere higher up in the build output? (One possible problem is there's a new dependency on libcurl, and I don't know if the Arch package reflects that yet.)
-
Thanks, fixed. (Technically it wasn't actually hanging forever, but you would have had to wait 41 years before it returned from the InitResolution() function.)
-
Hmm, odd. Could you try recompiling with "make CONFIG=Debug" (instead of CONFIG=Release) and then run "gdb ./pyrogenesis_dbg" (the _dbg is the new executable) and do exactly the same thing? And then (assuming it still hangs), after the "thread apply all bt full", type "fin" and press enter several times until it stops printing new messages?
-
The test failures are expected. The game shouldn't hang, though - perhaps you could run it in gdb to find out where it's stopping? Run the game with the command "gdb ./pyrogenesis" (assuming you've got gdb already - might need to do something like "port install gdb" if not), and it should say some stuff and then it should say "(gdb)", and then you should enter "r" (and press Enter), and wait a while for the game to start up (then it should hang like it did before), then do ctrl+c in gdb and enter "thread apply all bt full" and post the output from that.
-
Does it work better if you update to the most recent SVN (r8973 or later)?
-
Hmm, looks like it's including both of those files but then still failing to find the IID_AIManager that's defined in the second one. Still no idea why . Has anyone else ever had this problem, or is anyone else able to still compile successfully on OS X?
-
Hrm, sorry, I was sure I had replied to this, but the evidence suggests otherwise The initial error is In file included from ../../../source/simulation2/components/CCmpAIManager.cpp:21: ../../../source/simulation2/components/ICmpAIManager.h: In static member function static int ICmpAIManager::GetInterfaceId(): ../../../source/simulation2/components/ICmpAIManager.h:53: error: IID_AIManager was not declared in this scope and I don't know how that could happen The only thing I can think to try is: Edit source/simulation2/system/Component.h line 20 (immediately after the first "#define" line) and add "#warning XXXX". Edit source/simulation2/system/Components.h line 20 (immediately after the first "#define" line) and add "#warning YYYY". Compile again, and post the output. Hopefully that might indicate if it's somehow failing to pick up some of those files (which is the only explanation I can imagine).
-
Collecting data from users
Ykkrosh replied to Ykkrosh's topic in Game Development & Technical Discussion
That message means you're using an old version of the executable (from before SVN r8925) - maybe it had errors when you were compiling or something? -
Collecting data from users
Ykkrosh replied to Ykkrosh's topic in Game Development & Technical Discussion
I think this system is largely complete for now. It collects some more hardware details (like this - does anyone know of other values that would be useful to collect?) and profiler data (5 seconds and 60 seconds after launching a map, with the same data as pressing shift+F11 and looking in logs/profile.txt). The server needs more work to analyse the data nicely, but that doesn't need to be done right now (it could be after the next release if necessary). -
That definitely looks like an error, but not quite enough of it to understand it . Could you run the "make CONFIG=Release" command again and paste the whole output?
-
(Sorry, been a bit busy and didn't get time to reply to all this before now...) Added. The LatestReleaseMac page is about downloading the latest release (i.e. alpha 3). If you use SVN then that's later than the latest release, so you don't need to download anything more Could you try building with "make CONFIG=Release" (i.e. skip the "-j3"), and then upload the last dozen or so lines that it outputs? (It's possible that it's failing, but the error messages are hidden somewhere high in the output, because the "-j3" makes it continue a while after errors.) Hmm, that seems a lot of packages, and most of them aren't used directly by the game - they're probably used by other packages but in that case they should get installed automatically... Has anyone else had to explicitly install all these packages on OS X? (The list on the wiki worked for me some time ago.)
-
Collecting data from users
Ykkrosh replied to Ykkrosh's topic in Game Development & Technical Discussion
The important part is: In file included from ../../../source/ps/UserReport.cpp:23: ../../../source/lib/external_libraries/curl.h:50: fatal error: curl/curl.h: Datei oder Verzeichnis nicht gefunden which means you need to install libcurl - I think the package is "libcurl-dev" on Ubuntu. (Also I need to update the build instructions to mention this...) -
(I split these posts off from this thread.) This seems pretty weird... Has anyone else seen similar problems? I don't really have any idea what might cause it or how to go about trying to debug it
-
Collecting data from users
Ykkrosh replied to Ykkrosh's topic in Game Development & Technical Discussion
This page is an initial attempt at presenting a summary of some of the data. -
You could give him one of these
-
Collecting data from users
Ykkrosh replied to Ykkrosh's topic in Game Development & Technical Discussion
Currently it's like this.